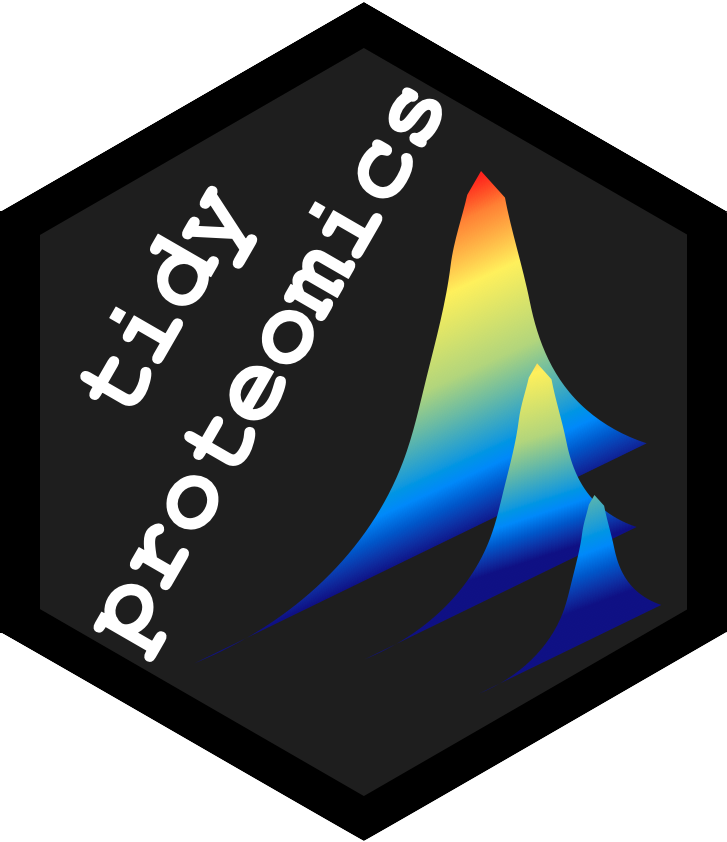
Compute protein enrichment
enrichment.Rd
enrichment()
is an analysis function that computes the protein summary
statistics for a given tidyproteomics data object.
Arguments
- data
tidyproteomics data object
- ...
two sample comparison e.g. experimental/control
- .pairs
a list of vectors each containing two named sample groups
- .terms
a character string referencing "term(s)" in the annotations table
- .method
a character string
- .score_type
a character string. From the fgsea manual: "This parameter defines the GSEA score type. Possible options are ("std", "pos", "neg"). By default ("std") the enrichment score is computed as in the original GSEA. The "pos" and "neg" score types are intended to be used for one-tailed tests (i.e. when one is interested only in positive ("pos") or negateive ("neg") enrichment)."
- .log2fc_min
used only for Fisher's Exact Test, a numeric defining the minimum log2 foldchange to consider as "enriched"
- .cpu_cores
the number of threads used to speed the calculation
- .significance_max
used only for Fisher's Exact Test, a numeric defining the maximum statistical significance to consider as "enriched"